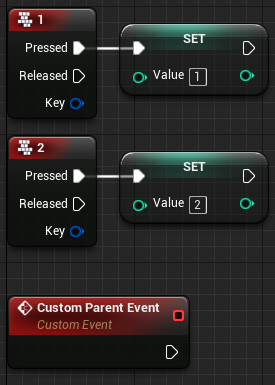
In this guide I will be going through Parent and Child blueprints in Unreal Engine 4. They are a key part of making manageable code and preventing repeated blueprint nodes in similar classes.
Inheritance is an important feature in many object oriented programming languages. It allows programmers to create variations of an object and communicate with them as if they are all the same original type.
Blueprints also have the ability to have child classes and have parent classes that they inherit from.
If you want to learn more about the theory behind inheritance, click here for a tutorial from w3 schools showing inheritance in C++.
Parent Class
Creating the Parent Class
To start, create a new blueprint actor and name it ParentActor.
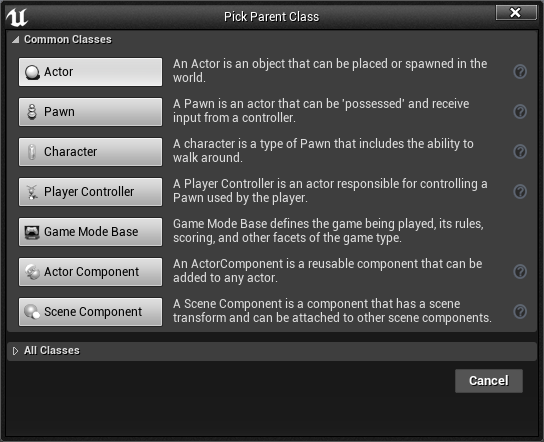
This actor can now be filled with events, input actions, variables, functions and more. All of these attributes will be shared to our child actors.
Setting up the Parent Class
To demonstrate, I have created two keybinds, an event, a function and a float variable.
Pressing 1 will set this float value to 1 and pressing 2 will set the float value to 2.
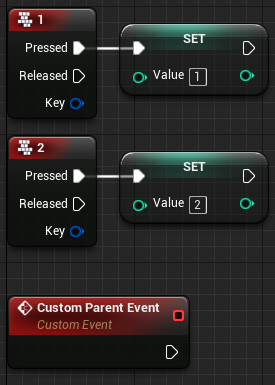
Child Class
Creating a child class
Creating a child class from your chosen parent class is very easy.
To create a new class based on a previous simply right click your chosen class in the content browser and click create child blueprint.
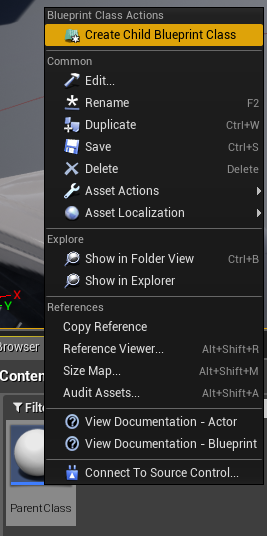
Accessing the parent
Variables
Inside the child actor there are no visible variables of the left side.
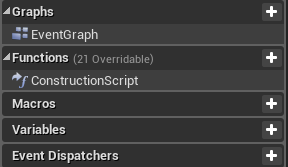
Right clicking and searching for your parent variables will reveal them in the default section.
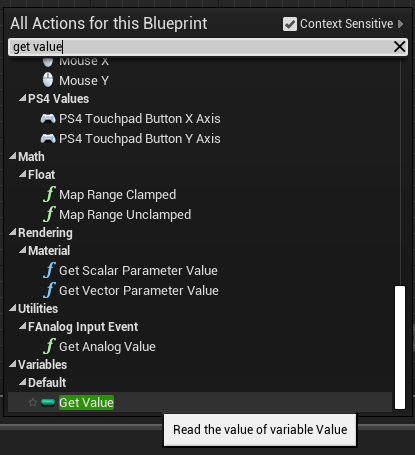
Events and Functions
Right clicking inside your child blueprint class and typing the name of your functions and events will give you access to them.
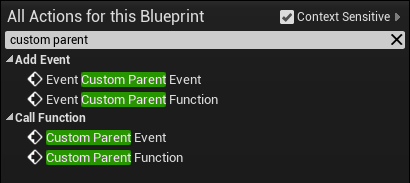
Key events will override any code that is on the same parent event. This child class will now print 10 on key press 1 and 20 on key press 2.
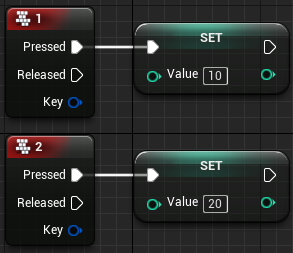
To make sure the parent code runs as well as the child code on these key inputs, click on the input and look to the right of the details panel.
Un-checking the “Override Parent Binding” box will run both sections of code. If you want your child to have different behaviors on the same key presses you can leave this checked.
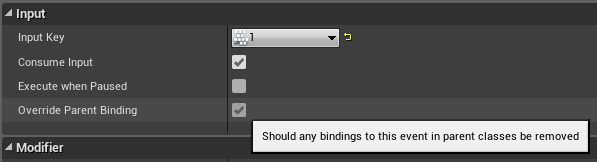
Running Parent Code
When accessing parent functions and events you are stopping the parent code from running. This is called overriding.
To make sure the parent code runs during the event, right click the specific event and click Add Call to Parent Function.
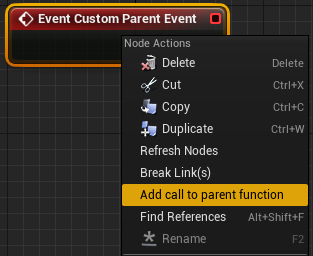

Now when custom parent event is fired the child code will run and the parent code will run. The expected output is “Hello From Parent Class” on screen.
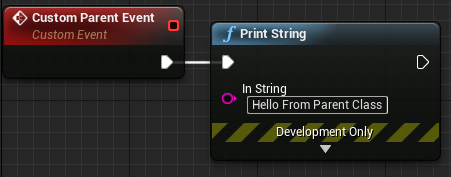
Conclusion
That is the basics of parent and child classes in unreal engine 4!
Designing your code to utilize this powerful technique will improve the readability and efficiency of your game development processes.
Leave a Reply