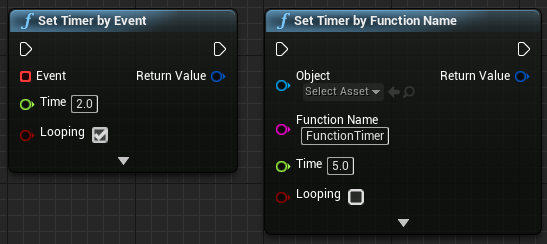
Timers are one of the best ways to run blueprint code periodically without using Event Tick. Unreal Engine will trigger your event or function automatically at the time set instead of constantly polling your functionality every frame.
Using timers can prevent actors reducing your game’s frame rate if implemented correctly.
In this guide we will be learning how to use Timers in Unreal Engine 5.
Prerequisites
To understand this guide and implement timers into your project, you will need to know how to use custom events and functions in Unreal Engine 5 before starting.
Click here to read our guide on custom events.
How to create Blueprint Timers
Basic Timer Settings
Before we create our timer, we will look at the basic timer settings that all timers have. These allow us to set the frequency of the timer and if the timer repeats or not.
The green Time pin is the time Unreal Engine will wait to call your event. If this value is less than or equal to 0, the timer will be cleared and will not call the event.
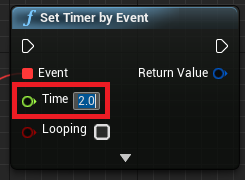
The Looping check box will repeat the timer if set to true and only run once if set to false. Ticking this box is best for functionality that you want to run repeatedly.
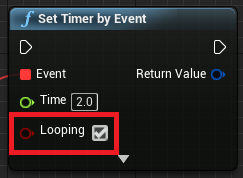
Timer by Event
When you want to create a timer that uses a custom event in the event graph, you need to use Set Timer by Event.
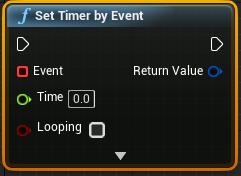
As shown below, your custom events can be connected using the red event delegate square. Any custom event are connected to this pin to trigger on the time set. Only one event is connected at a time.
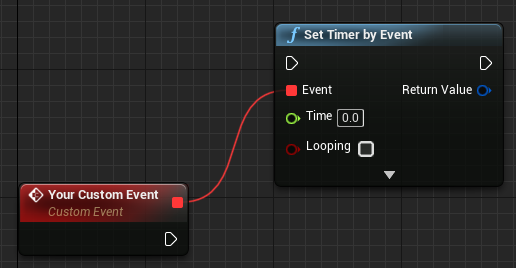
Timer by Function
If you want to use a function instead of an event delegate, we have the Timer by Function Name blueprint node. This has function name and object pins replacing the red delegate pin.
Object is the reference to the object that you want to run the function on (e.g. FirstPersonCharacter or your own custom actors). If you want to run the function on this actor, you can leave this pin empty.
“Function Name” is the name of the function found in the function list in your object.
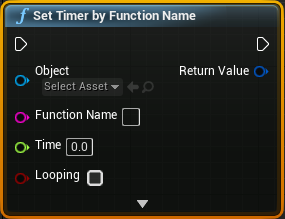
In this example we have created a new function in the My Blueprint panel on the left of the blueprint editor. Our new function is called MyCustomFunction.
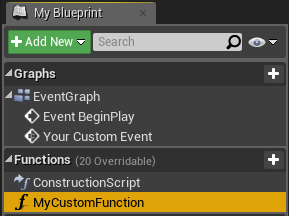
Now our function has been created, we then add the functions name into the Function Name pin.
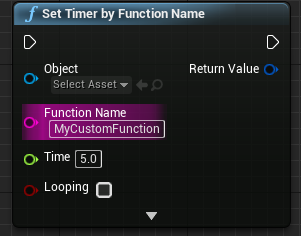
The blueprint node above now run MyCustomFunction after 5 seconds without looping.
Saving the Timer
If you want to change any properties about a timer, you need to save a reference to the return value. The easiest way to do this is to drag from the return value and click Promote to Variable.
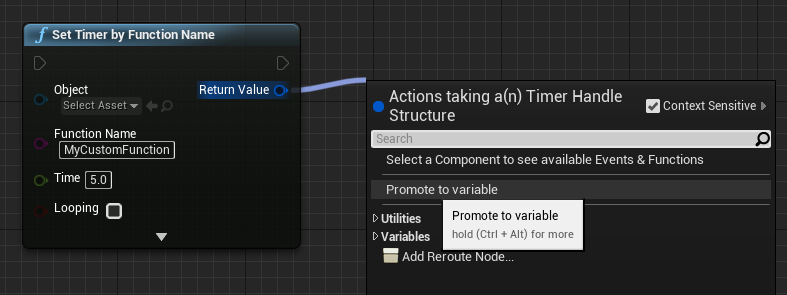
Pausing and Unpausing Timers
Now we have a reference to our timer, we can pause and un-pause the timer. The nodes below show the handle and function versions of pausing and unpausing your timer.
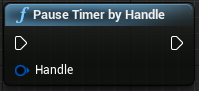
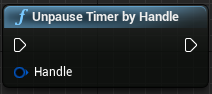
Function timers can be paused and unpaused using the function name and do not need a timer handle.
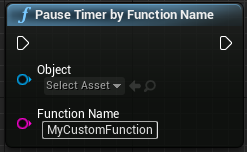
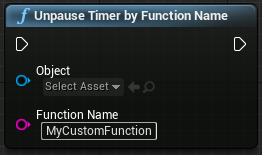
Removing a Timer
When you need a timer to stop, use the clear and invalidate timer blueprint nodes. These nodes will stop the timer and destroy the specified handle object.
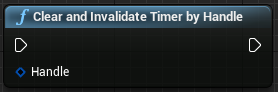
When using a Function Timer, you will need to clear and invalidate the timer separately if you want to use a function name.
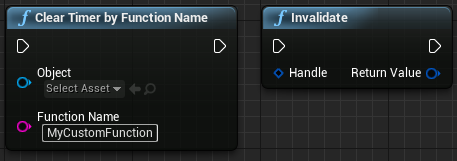
Checking a Timer’s Status
With the handle or function name, you can check various properties about a timer.
Firstly we have Does Timer Exist. These nodes check if the timer exists in memory. If the timer is cleared and invalidated this value is false.
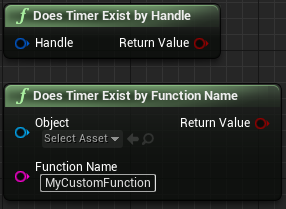
Is Timer Active will return true if the handle or function name has a timer that is actively running and exists.
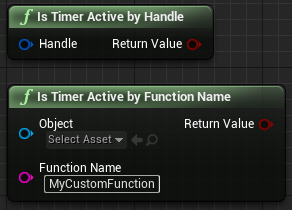
“Is Timer Paused” returns true if the timer is not actively running but does exist.
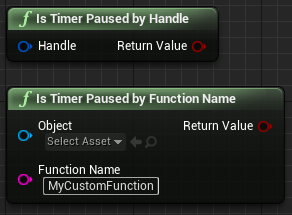
Conclusion
Now you have all the knowledge to use timers in your blueprint code in Unreal Engine 5!
Having all of these nodes at your disposal allows code and functionality to run at specific times and at certain frequencies. This will overall improve the readability of your code and can bring great performance gains. We recommend setting the Time value to 0.1 or 0.05 seconds instead of running every frame on tick.
Further reading:
In depth use of timers with gameplay example from Unreal Engine documentation
Click here to read how C++ Timers work in Unreal Engine
Leave a Reply