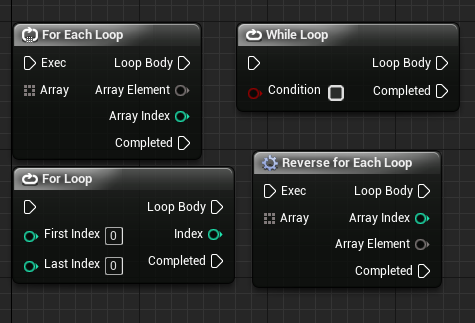
When creating games you will always need some way to store multiple pieces of information together. Luckily every game engine already have this covered. In this guide I will show you how to use loops and arrays in Unreal Engine 4.
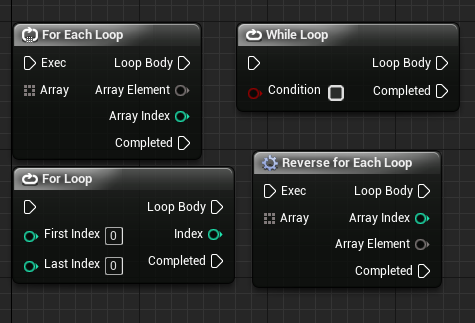
In Unreal you can create Arrays which give you the ability of storing as many pieces of information together within one variables as long as they are the same variable type (Float, Integer, Vector, Actor, etc.).
A few real game uses for arrays are storing items inside their backpack and location way points for your AI characters to navigate to.
Loops are used to navigate through your arrays and access individual data. This then can be used to effect your game based on this data.
Arrays
What is an Array?
An array is a sequence of objects that are all of the same type.
For a further theory dive into arrays using C++ click here.
Creating an Array
Firstly create a variable of the type you want. In this case I used a float.
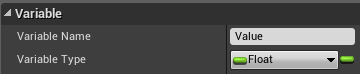
Create the variable, press the button to the side of the variable type then select the array button to convert this variable to an array.
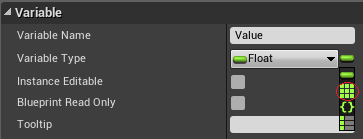
Populating the Array
Now you can populate your array with the plus button. Separate floats have now been created and can be changed independently of each other.
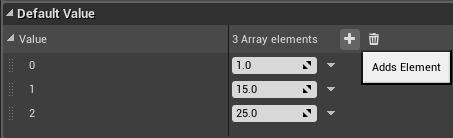
Your array is now fully setup!
Accessing your Array
Below are the main blueprint nodes that you will be using when accessing your arrays. For the complete list of array nodes click here.
Accessing a specific index in your array uses the GET node:
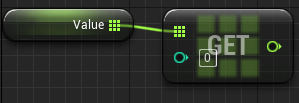
To add an element to the end of your array use the ADD node:
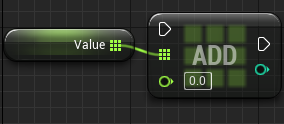
Removing a specific index in your array uses the REMOVE INDEX node:
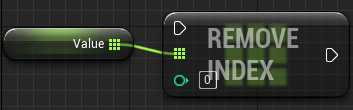
Setting a specific array element to a new value uses the SET ARRAY ELEM node:
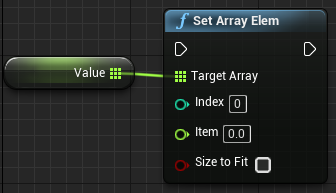
To check if your array contains a specific element/value use the FIND ITEM node:
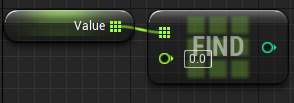
When you need to remove all array elements use the CLEAR node:
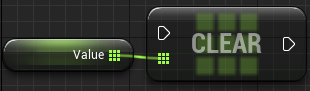
Loops
Loops iterations are slow to process so use them sparingly!
While Loop
The simplest type of loop is the While Loop. This executes while the input Boolean is true.
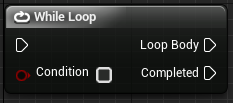
Be careful when using While Loops as they can easily cause the engine to detect and infinite loop. Make sure to use delays inside the loop to prevent the maximum iteration counter from stopping your game running.

For Loop
The next type of loop is the For Loop. This will execute your desired blueprint nodes X amount of times based on your settings.

The ForLoop node gives you the choice of which index to start at and which to end at. The loop will execute and add 1 to the index value, returning this value in the index pin on the right.
Loop body is the pin in which you connect the code you wish to loop through.
Once your loop has finished the completed pin will execute.
For Loop with Break
The For Loop with Break node gives you the option to end your loop early if required. This is especially useful when optimising loops as many loops don’t need to be fully run through if your code has done what it needs to do.
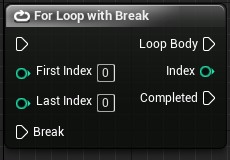
For Each Loop
The for each loop takes an array as an input and gives easy access to every element inside such array.
For Each Loops are especially slow to process in blueprints compared to C++ so try not to use them very often in your blueprint classes.
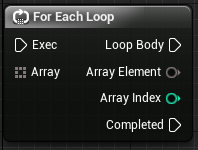
When working with your arrays, these are the most useful as it gives easy access to current array elements and its respective index.
For Each with Break
The for each node has a variety called for each with break. This gives you the option, similar to the For Loop with Break, to end the loop at a certain point if your required condition is met.
An example of this is looking for a specific item inside your player’s inventory.
You would loop through every index until you found a stack of this item, check if this stack is not full then break the loop as no more processing is required.
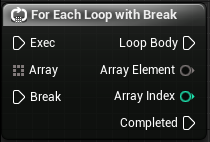
Reverse For Each Loop
The last type of For Each loop is the reverse for each loop. This differs from the original loop by starting at the end of the array and working backwards towards the start.
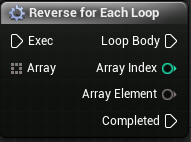
Conclusion
Now you have the knowledge on how to use arrays and loops in Unreal Engine 4!
For a more detailed breakdown of flow control and the blueprint nodes featured in this guide click here for the Unreal Engine documentation page.
Leave a Reply