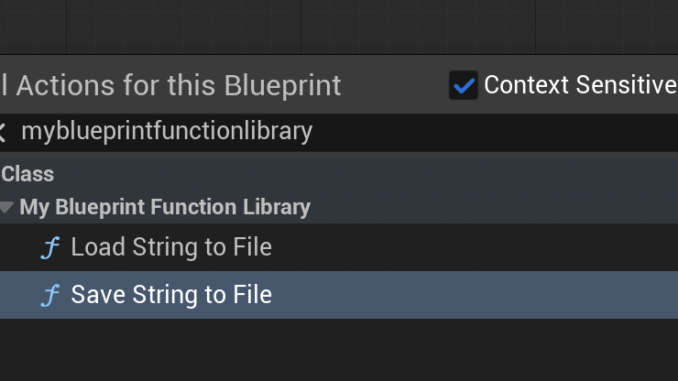
In every game development project there will come a time where your Blueprint code is running too slow and can’t be optimised any further.
Writing your code entirely in C++ will always run faster but requires a complete restructure of your project to easily work with your existing Blueprint code.
Also, there are C++ features you may want to use in your project that do not have Blueprint nodes available.
Using a C++ Function Library, we can gain the functionality, speed, and efficiency of C++ code while integrating effortlessly into your existing blueprint code!
In this guide we explain how to use C++ Blueprint Function Library in UE5. This contains what a C++ Blueprint Function Library is, how to create one in your project, and how to access your function library in your blueprint editor.
Prerequisites
This guide expects a basic level of knowledge of C++ in Unreal Engine 5.
To follow this guide you also need to have one of the following:
- Visual Studio for Windows
or - XCode for MacOS
These programs are used to compile the C++ code into your project. If you do not have a IDE installed, the engine will prompt you to install one.
What is a C++ Blueprint Function Library
The C++ Blueprint Library is a built in Unreal Engine class that allows code to be written using the C++ programming language to be used in both C++ code or in Blueprints!
This means we can move our slow blueprints nodes into optimised C++ code then run these functions inside of our blueprint code.
This gives us the power of C++ inside of blueprints in an easily accessible form!
How to create a C++ Blueprint Function Library
C++ Project vs Blueprint Project
Firstly, we need to find out if our project is a C++ enabled project, or only a blueprint enabled project. Luckily this is easy to do!
Click the Tools drop down at the top of the screen, then click “New C++ Class“.
If you do not have one of the preqrequisite programs installed as explained earlier, the engine will instruct you what you need to install.
In our case, for this guide, we used a MacOS machine, and so we installed XCode.
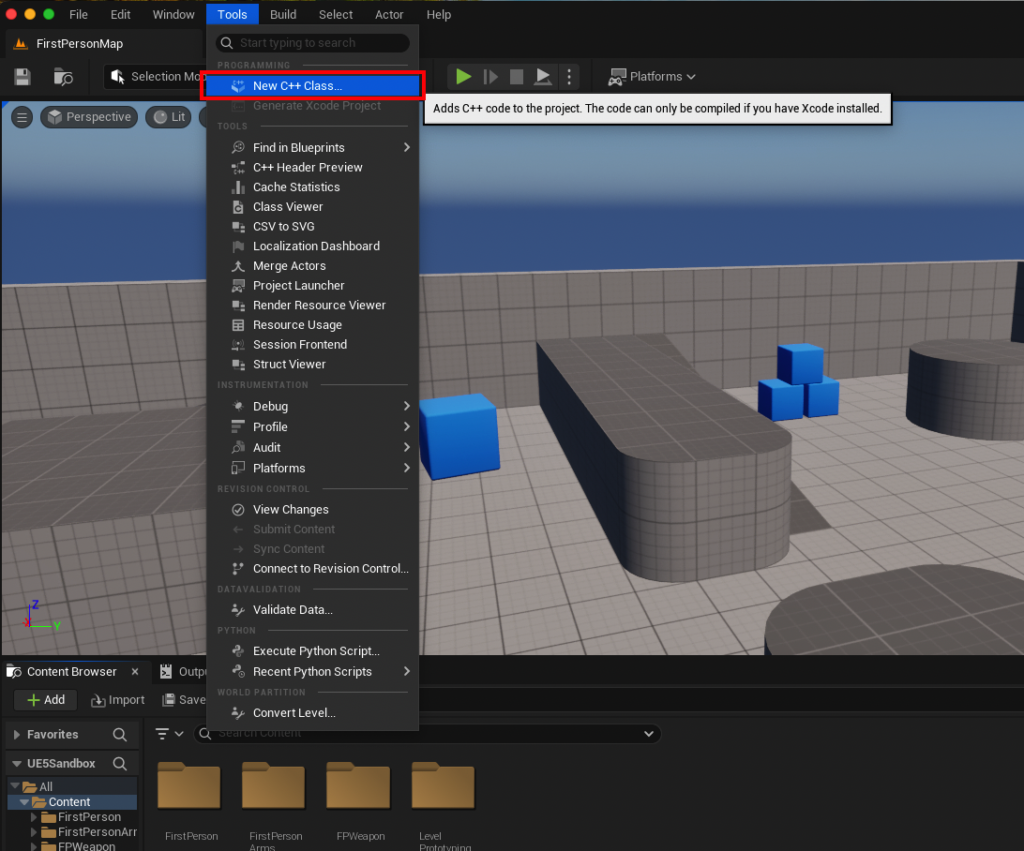
Next, the dialog box will appear asking which type of “Parent Class” you wish to use when creating your new class.
As we are making a C++ blueprint function library, we scroll down near the bottom and click “Blueprint Function Library”. To move to the next section, we press the blue NEXT button.
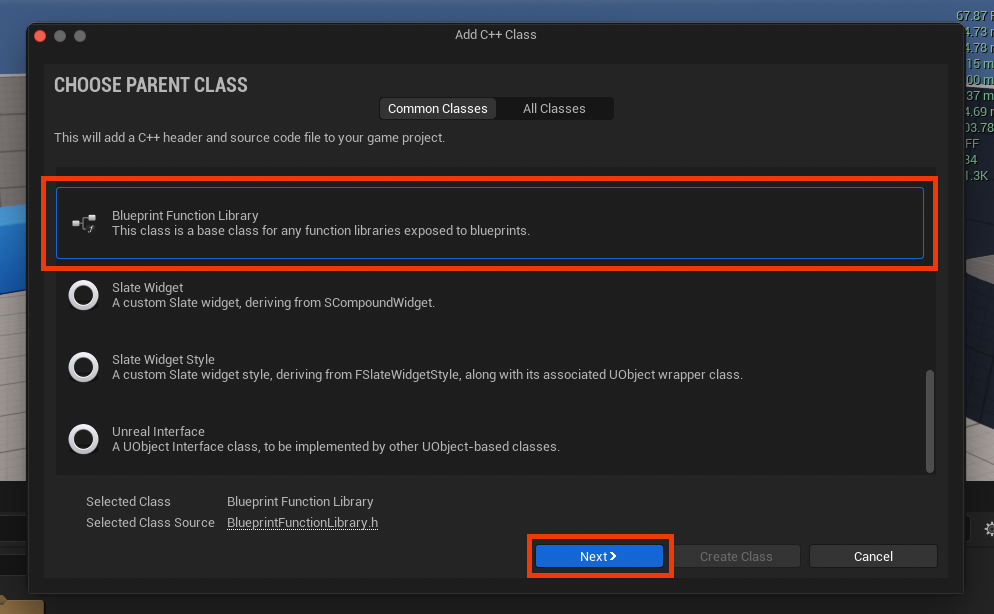
We then are asked to choose a name for our new blueprint function library class.
In this guide we used the default name “MyBlueprintFunctionLibrary”. You can change this to your choice of name.
We recommend a clear name with capital letters for each word in the name to follow Unreal Engine 5’s naming convention.
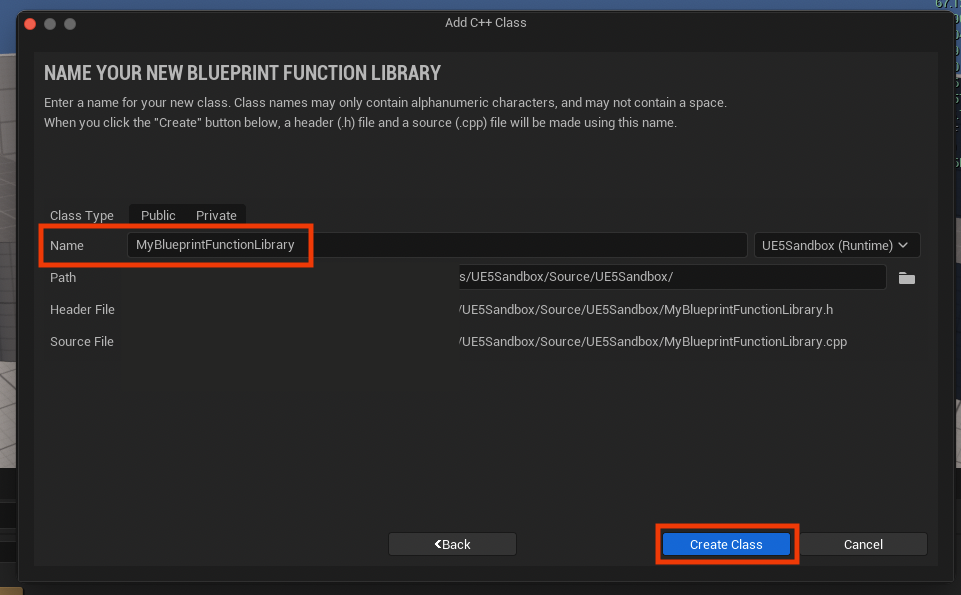
After naming our class and clicking the Create Class button, our project then compiles the new files for us to use. Wait for this to complete before moving on the next section.
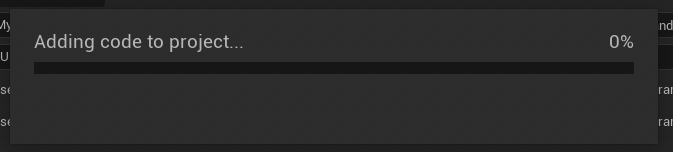
Inside your Code IDE
Now that our new class has been created, we can now open our IDE of choice and look at the files Unreal Engine has created for us.
As we chose “MyBlueprintFunctionLibrary” as our class name, we can see that we have two files. A .cpp file for our code, and a .h header file for our declarations both with our chosen name.
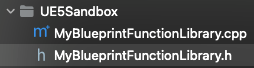
Add functions
Now we are ready to add our C++ code to add functionality to our Blueprint Function Library.
For this guide we are making a simple read and write system for strings. This will take our string and save it to a specified file, and can also read a file into a Unreal Engine string.
For your project, you can write the C++ code necessary for your required features.
First in our header file we can see that the engine has generated us a basic file with the correct includes and inheritance for our class.
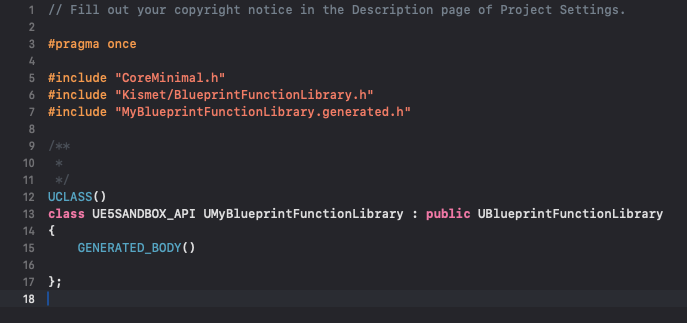
Next we add our function declaration. We have added a basic function that returns a true or false bool based on if we can save the string to the file or not.
The most important part is to use the static keyword. This makes our function accessible anywhere without an object reference!
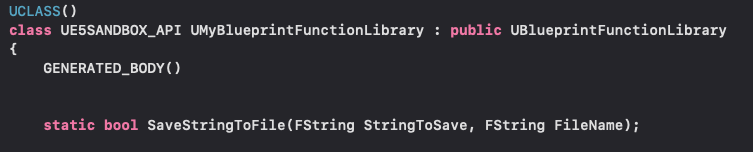
We then must add our UFUNCTION macro to make our function visible inside of our blueprint editor.
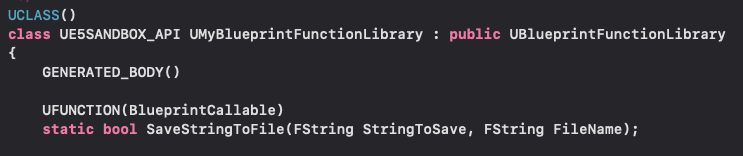
Next, we repeat the same as before with our loading string from file function. As before it is static with a UFUNCTION macro for blueprint compatibility.
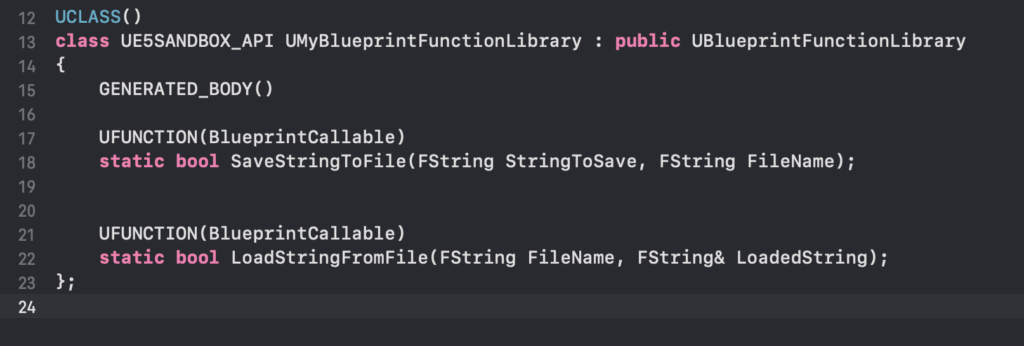
Lastly, we open the .cpp file and add our function code.
This is a simple use of the FFileHelper library in Unreal Engine 5 to allow us to read and write strings to files.
Our Blueprint Function Library is now a powerful example of what we can do when we need access to C++ functionality inside of our blueprints!
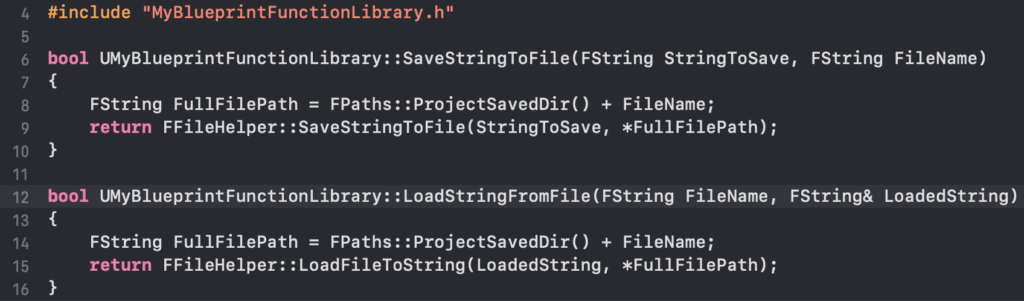
Once you have completed this step, compile your project and relaunch the editor.
How to use the C++ Blueprint Function Library in Blueprints
Finally, we can open any blueprint editor and type the name of our new blueprint function libary.
We can see in the actions pop up box that our two “Load String to File” and “Save String to File” C++ functions are now exposed to the blueprints system!
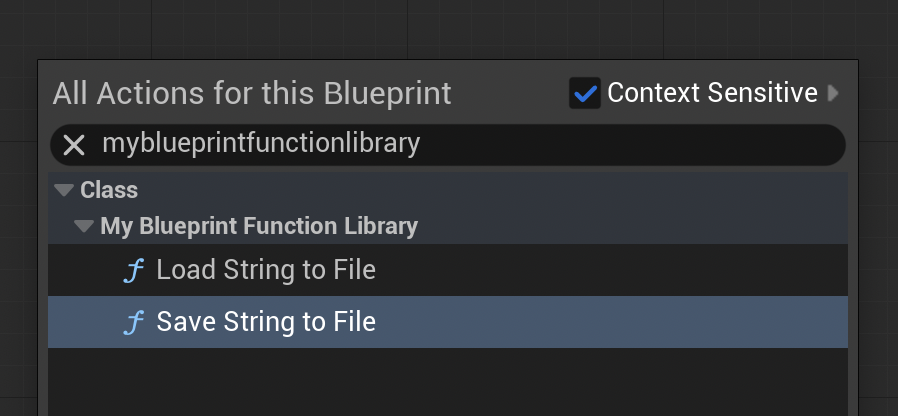
Demonstration
To demonstrate our functions working, we opened the level blueprint, created a BeginPlay event, and connected our new Save String to File C++ Blueprint Library function to it.
We can specify any string to save, and choose a file name.
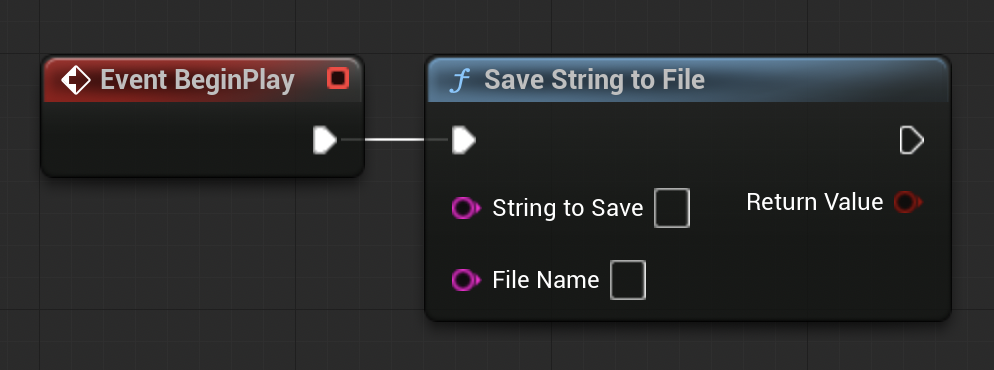
After running this with “Save Test” as the String to Save and textfile-test.txt as the File Name, we can see in the project /Saved folder our file and string!
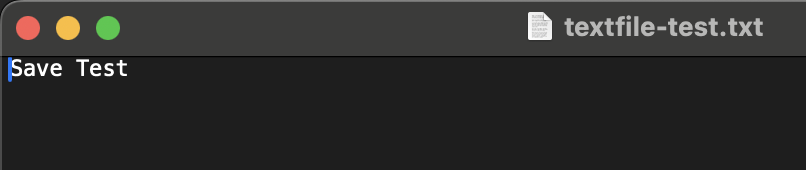
Lastly, we can test our Load String from File function inside of the editor the same way.
We have created the loadtest.txt file in the /Saved folder of our project, and written the string “Loading Test” into it and saved.

Inside of the level blueprint we then add our Load String from File C++ Blueprint Function Library function and connected it to a print string node.
This will load of text file into a string and print it to the screen.
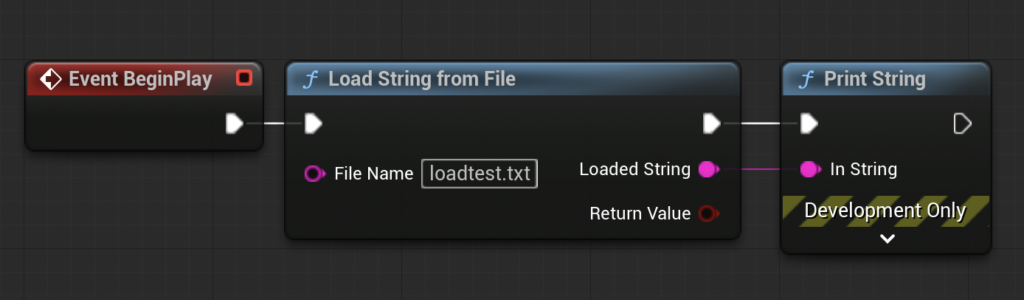
As you can see, after playing our project in the editor, our string from inside our file is show on screen!
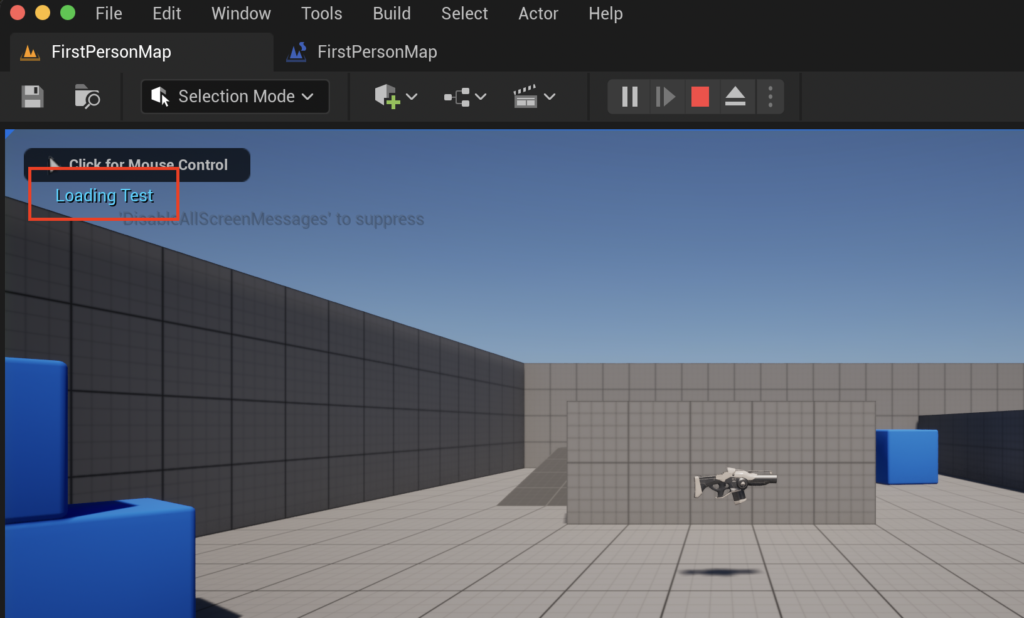
Conclusion
Now you understand how to use C++ Blueprint Function Libraries in Unreal Engine 5!
The use of these function libraries gives tangible performance improvements and also unlocks the hidden functionality inside of the engine; exposing this to your blueprint code!
Further Reading
Official blueprint function library documentation from Epic Games
Click here to read more of our C++ guides for Unreal Engine
Leave a Reply